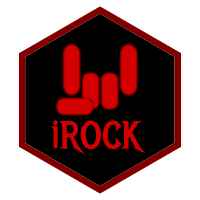
Export parsed sources to HTML or Markdown
Source:R/export_to_html.R
, R/export_to_markdown.R
exporting_sources.Rd
These function can be used to convert one or more parsed sources to HTML, or to convert all sources to tabbed sections in Markdown.
Usage
export_to_html(
input,
output = NULL,
template = "default",
fragment = FALSE,
preventOverwriting = rock::opts$get(preventOverwriting),
encoding = rock::opts$get(encoding),
silent = rock::opts$get(silent)
)
export_to_markdown(
input,
heading = "Sources",
headingLevel = 2,
template = "default",
silent = rock::opts$get(silent)
)
Arguments
- input
An object of class
rock_parsedSource
(as resulting from a call toparse_source
) or of classrock_parsedSources
(as resulting from a call toparse_sources
.- output
For
export_to_html
, either NULL to not write any files, or, ifinput
is a singlerock_parsedSource
, the filename to write to, and ifinput
is arock_parsedSources
object, the path to write to. This path will be created with a warning if it does not exist.- template
The template to load; either the name of one of the ROCK templates (currently, only 'default' is available), or the path and filename of a CSS file.
- fragment
Whether to include the CSS and HTML tags (
FALSE
) or just return the fragment(s) with the source(s) (TRUE
).- preventOverwriting
For
export_to_html
, whether to prevent overwriting of output files.- encoding
For
export_to_html
, the encoding to use when writing the exported source(s).- silent
Whether to suppress messages.
- heading, headingLevel
For
Examples
### Get path to example source
examplePath <-
system.file("extdata", package="rock");
### Parse a selection of example sources in that directory
parsedExamples <- rock::parse_sources(
examplePath,
regex = "(test|example)(.txt|.rock)"
);
### Export results to a temporary directory
tmpDir <- tempdir(check = TRUE);
prettySources <-
export_to_html(input = parsedExamples,
output = tmpDir);
### Show first one
print(prettySources[[1]]);
#> [1] "\n<html><head>\n\n<style>\n\n\n.code {\n border-radius: .25em;\n box-sizing: border-box;\n margin-left: 10px;\n padding: 2px;\n}\n\n.codeValue {\n border-radius: .25em;\n box-sizing: border-box;\n margin-left: 10px;\n padding: 2px;\n}\n\n.identifier {\n border-radius: .25em;\n box-sizing: border-box;\n margin-left: 10px;\n padding: 2px;\n}\n\n.sectionBreak {\n border-radius: .25em;\n box-sizing: border-box;\n display: block;\n}\n\n.uid {\n border-radius: 0;\n box-sizing: border-box;\n}\n\n.utterance {\n display: block;\n}\n\n/*\n\n.code {\n background-color: #457BB7;\n}\n.networkCode {\n background-color: #C56A33;\n}\n\n.codeValue {\n background-color: #DBA42D;\n}\n\n.identifier {\n background-color: #C27FAB;\n}\n\n.sectionBreak {\n background-color: #eeeeff;\n}\n\n.uid {\n background-color: #eeeeee;\n color: #bbbbbb;\n}\n\n.utterance {\n border-top: 1px dotted black;\n border-bottom: 1px dotted black;\n}\n\n.utterance + .utterance {\n margin-top: -1px;\n}\n\n*/\n\n/* Now handled with opacity /*\n/*.context {\n color: #bbbbbb;\n} */\n\ndiv.rock-collected-fragments-container {\n margin: 10px;\n}\n\ndiv.rock-code-heading {\n margin: 30px 5px 5px 5px;\n}\n\ndiv.rock-code-heading em {\n color: #aaaaaa;\n}\n\n.rock-sought-codeIdentifier {\n font-weight: bold;\n}\n\n\n/* - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - -*/\n\n.rock-source-filename {\n background-color: #ffffee;\n color: #cccc66;\n margin: 3px 20px 8px 20px;\n padding: 2px 10px;\n border: 1px solid #cccc66;\n}\n\n.rock-source-filename pre {\n display: inline;\n background-color: #ffffee;\n color: #cccc66;\n}\n\n\n.sectionBreak {\n background-color: #ddddff;\n color: #888899;\n text-align: center;\n}\n\n.rock-yaml-chunk {\n background-color: #ddffdd;\n color: #55aa55;\n}\n\ndiv#codableSource {\n font-family: 'Ubuntu Mono', monospace;\n font-weight: bold;\n font-size: 16px;\n box-sizing: border-box;\n width: 100%;\n max-width: 100%;\n padding: 10px;\n border: 2px solid black;\n border-radius: 10px;\n background-color: #fff;\n}\n\ndiv.rock-line {\n min-height: 1.1em;\n line-height: 1.1em;\n font-family: 'Ubuntu Mono', monospace;\n font-size: 14px;\n}\n\ndiv.rock-utterance {\n font-weight: bold;\n border-bottom: 1px dotted #aaa;\n border-top: 1px dotted #aaa;\n cursor: crosshair;\n min-height: 1.8em;\n line-height: 1.9em;\n}\n\n/* If the next element is also an utterance, remove the bottom-line,\n since it will have a top-line already */\n\ndiv.rock-utterance:has(+ div.rock-utterance) {\n border-bottom: 0px;\n}\n\n.rock-comment {\n font-weight: normal;\n color: #999;\n cursor: auto;\n}\n\n.rock-coding {\n padding: 3px;\n border-radius: 3px;\n cursor: not-allowed;\n margin: 2px;\n}\n\nhr.rock-fragment-delimiter {\n border: 0;\n height: 1px;\n background-image: linear-gradient(to right, rgba(0, 0, 0, 0), rgba(0, 0, 0, 0.75), rgba(0, 0, 0, 0));\n\n overflow: visible; /* For IE */\n padding: 0;\n border: none;\n /* border-top: medium double #333;\n color: #333; */\n text-align: center;\n\n}\n\n/* From https://css-tricks.com/examples/hrs/ */\n/* Specifically, this trick is called Glyph, by Harry Roberts */\n\nhr.rock-fragment-delimiter-within:after {\n content: \"§\";\n display: inline-block;\n position: relative;\n top: -0.8em;\n font-size: 1.2em;\n padding: 0 0.25em;\n background: white;\n}\n\n\nhr.rock-fragment-delimiter-above:after {\n content: \"start\";\n display: inline-block;\n position: relative;\n top: -0.8em;\n font-size: 1.2em;\n padding: 0 0.25em;\n background: white;\n}\n\nhr.rock-fragment-delimiter-below:after {\n content: \"end\";\n display: inline-block;\n position: relative;\n top: -0.8em;\n font-size: 1.2em;\n padding: 0 0.25em;\n background: white;\n}\n.addCodeButton .rock-coding {\n cursor: crosshair;\n}\n\n.rock-uid {\n background-color: #eee;\n color : #aaa;\n}\n\n.rock-ciid {\n background-color: #c27fab;\n color : white;\n}\n\n.rock-treeCode {\n background-color: #457bb7;\n color : white;\n}\n\n.rock-codeValue {\n background-color: #dba42d;\n color : white;\n}\n\n.rock-networkCode {\n background-color: #c56a33;\n color : white;\n}\n\n.context {\n opacity: .5;\n}\n</style>\n\n</head><body>\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">This file is a very drafty file used to test stuff. It contains some meeting minutes and some other stuff.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">The contents aren't relevant; it's all about the parsing of the ROCK conventions.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Coding tree specifications:</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"> Coding trees are by definition hierarchical: there is a root with children (potentially with children of their own, and so on, with arbitrary depth). Every node in such a tree has at least an identifier, which can contain only lowercase letters, nummers, and underscores. In addition, it can have a label, which can be anything and is the human-readable name, and a code which is used to code the utterances. If a label or code is not specified, the identitier is used (for example, if the identifiers are suitable for coding, it will often not be necessary to specify both identifiers and codes; but it is possible to enable use of DCT identifiers as identifier while using codes that are more practical). Nodes can optionally have one or more children. Since only the identifier is mandatory (and copied to codes and labels if those are omitted), children can be specified using a shorthand using a list of identifiers contained between square brackets and separated by comma's.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Stanzas are not necessarily higher-level codes.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Stanzas are sets of utterances within which you want to look for code co-occurrence.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">People's narratives convey information about their psychology. In their narratives,</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Co-occurrences</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Utterances Sections Stanzas StanzaType Strophes</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">---------------------------------------------------</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">1 1 1 A 1</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">2 1 1 A 1</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">3 1 2 B 2</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">4 1 3 A 1</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">5 2 3 A 1</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Utterance = smallest unit of analysis, usually one sentence, in any case, one line (a line being defined as a set of characters ending with a line ending)</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Section = a set of one or more utterances that follow each other and fall between the same two section markers ('nongreedy')</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Stanza = a set of one or more utterances that occur in close proximity and that are defined by ... (the complicated bit), i.e. the smallest unit of analysis psychologically. Stanzas are a solution for the fact that peoples' sentence length is not closely related to the psychological unity of the matter they discuss. E.g. Alice could discuss her ideas about the cause of the disease in three sentences while Bob would use only one, but both explanations would be the same stanza. Stanzas are what in written text would be paragraphs. Except that spoken text is more messy, and so 'paragraphs' can be interspersed by unrelated utterances. Therefore, utterances in close proximity can be combined into the same stanza even if they are separated by a small number of unrelated utterances. Note, however, that stanzas remain defined as 'messy paragraphs'; if an interviewee starts referring back to something discussed half an hour earlier, that doesn't justify combining those utterances into the same stanza. (Note, however, that they _could_ be combined through stanza sets / strophes, if they happen to be codes by the same codes, _and_ if strophes are composed by collapsing stanzas with those codes.)</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Strophe = a set of one or more stanzas that are combined based on sharing the same code, identifier, or attribute. Strophes, therefore, are collected over the entire transcript/source or over all transcripts/sources; proximity is irrelevant.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Conversation = a transcript = a source</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Case = a person (assuming persons are the research unit, could in theory also be an organisation etc)</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Attribute = a characteristic of a case (that can then be designated to utterances as well)</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Unit = a set of one or more utterances that share a given attribute</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Discourse = all utterances</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Topic = a subject area discussed in the interview - i.e. topics are known beforehand and together form the interview scheme / questionnaire / whatever</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<paragraph_break>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Why did you do that? <span class=\"rock-coding rock-treeCode code codes\">[[Topic1]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span> <span class=\"rock-ciid identifier ciid\">[[tid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<B>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<A>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. <span class=\"rock-coding rock-treeCode code codes\">[[inductMother>inducChild1]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged.<span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild3]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum. <span class=\"rock-coding rock-treeCode code codes\">[[inductMother>inducChild2]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Why do we use it? <span class=\"rock-coding rock-treeCode code codes\">[[inductMother>inducChild1]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout. <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild4]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<A>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">The point of using Lorem Ipsum is that it has a more-or-less normal distribution of letters, as opposed to using 'Content here, content here', making it look like readable English. <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Many desktop publishing packages and web page editors now use Lorem Ipsum as their default model text, and a search for 'lorem ipsum' will uncover many web sites still in their infancy. <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild3]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<A>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<B>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Where does it come from? <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild4]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Contrary to popular belief, Lorem Ipsum is not simply random text. <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild5]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<paragraph_break>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">It has roots in a piece of classical Latin literature from 45 BC, making it over 2000 years old. <span class=\"rock-coding rock-treeCode code codes\">[[Topic2]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span> <span class=\"rock-ciid identifier ciid\">[[tid=2]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Richard McClintock, a Latin professor at Hampden-Sydney College in Virginia, looked up one of the more obscure Latin words, consectetur, from a Lorem Ipsum passage, and going through the cites of the word in classical literature, discovered the undoubtable source. <span class=\"rock-coding rock-treeCode code codes\">[[tables]]</span> <span class=\"rock-coding rock-treeCode code codes\">[[people]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Lorem Ipsum comes from sections 1.10.32 and 1.10.33 of \"de Finibus Bonorum et Malorum\" (The Extremes of Good and Evil) by Cicero, written in 45 BC. <span class=\"rock-coding rock-treeCode code codes\">[[oaken_chests]]</span> <span class=\"rock-coding rock-treeCode code codes\">[[people]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">This book is a treatise on the theory of ethics, very popular during the Renaissance. <span class=\"rock-coding rock-treeCode code codes\">[[people]]</span> <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">The first line of Lorem Ipsum, \"Lorem ipsum dolor sit amet..\", comes from a line in section 1.10.32. <span class=\"rock-ciid identifier ciid\">[[cid=1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\"><span class=\"rock-coding sectionBreak sectionBreak\">---<<A>>---</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">The standard chunk of Lorem Ipsum used since the 1500s is reproduced below for those interested. Sections 1.10.32 and 1.10.33 from \"de Finibus Bonorum et Malorum\" by Cicero are also reproduced in their exact original form, accompanied by English versions from the 1914 translation by H. Rackham.</div>\n</div>\n\n<div class='utterance'><div class=\"rock-line \"><span class=\"rock-comment\"></span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">Where can I get some? <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild3]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form, by injected humour, or randomised words which don't look even slightly believable. <span class=\"rock-coding rock-treeCode code codes\">[[chairs]]</span> <span class=\"rock-coding rock-treeCode code codes\">[[internet]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">If you are going to use a passage of Lorem Ipsum, you need to be sure there isn't anything embarrassing hidden in the middle of text. <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild3]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">All the Lorem Ipsum generators on the Internet tend to repeat predefined chunks as necessary, making this the first true generator on the Internet. <span class=\"rock-coding rock-treeCode code codes\">[[internet]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">It uses a dictionary of over 200 Latin words, combined with a handful of model sentence structures, to generate Lorem Ipsum which looks reasonable. <span class=\"rock-coding rock-treeCode code codes\">[[inductFather>inducChild4]]</span> <span class=\"rock-coding rock-treeCode code codes\">[[inductMother>inducChild1]]</span></div>\n</div>\n\n<div class='utterance'><div class=\"rock-line rock-utterance utterance\">The generated Lorem Ipsum is therefore always free from repetition, injected humour, or non-characteristic words etc. <span class=\"rock-coding rock-treeCode code codes\">[[tables]]</span> <span class=\"rock-coding rock-treeCode code codes\">[[att_ins_eval]]</span></div>\n</div>\n\n</body></html>\n"